Since OpenAI’s GPTs were launched, AI chatbots have taken on all kinds of tasks, from helping plan weekly meals to managing customer complaints for big businesses. These chatbots are proving to be super helpful in automating time-consuming tasks, both in our personal lives and at work. But how do you actually build an AI chatbot using Python and NLP?
In this blog, we’ll walk you through the step-by-step process of creating a simple conversational AI chatbot using Python and NLP.
Understanding AI Chatbot
An AI chatbot is a smart software that can mimic human conversation, whether through text or voice.

Thanks to artificial intelligence, especially natural language processing (NLP), these chatbots can understand and reply to users in a way that feels natural and human-like. They’re becoming more common in industries like customer service, e-commerce, and more, offering help 24/7, answering customer questions, and even supporting sales and marketing efforts.
AI chatbots are designed to learn from each conversation, so they get better at responding over time and can provide a more personalized experience. By integrating them into business operations, companies can boost customer engagement, cut down on costs, and make their processes more efficient.
Different types of AI chatbots
Before you start building your own AI chatbot, let’s first take a look at the different types of chatbots out there.
Rule-based Chatbots
Rule-based chatbots follow a set of predefined rules, so their conversations are all scripted. These are great for simple tasks, like providing pre-written answers or following specific instructions. However, they can’t think outside the box and aren’t good at handling more complex or nuanced conversations.
AI-powered Conversational Chatbots
AI-powered chatbots use advanced technology to carry on conversations in a way that feels more human. They learn from past interactions, can pull information from documents, and even handle multiple languages. These chatbots are perfect for a variety of tasks, from answering common questions to handling more complicated product inquiries.
Each type of chatbot has its own strengths, so the right choice depends on what your business needs and aims to achieve.
The Smarter Way to Build AI Chatbot
Alltius is a GenAI platform that lets you create smart, secure, and accurate AI assistants without any coding. With Alltius, you can set up your own AI assistant in just a few minutes, using your own documents.
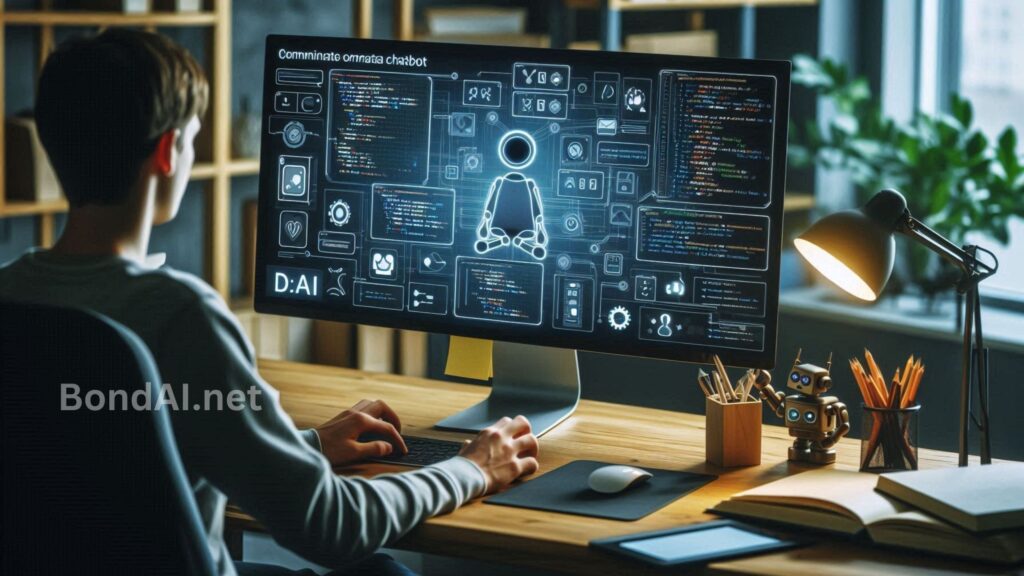
What makes Alltius stand out is the wide range of data sources you can use to train your AI assistant. Whether it’s PDFs, videos, emails, images, Excel sheets, APIs, web pages, FAQs, or more, Alltius can handle it. Once trained, your AI assistant can greet visitors, answer questions, pull information from documents, write pitches, draft emails, extract insights, and much more. Plus, it can be deployed on your website, Slack, Zendesk, Intercom, or even your product.
Let’s break down how easy it is to create your own conversational AI assistant with Alltius.
Step 1: Create Your AI Assistant
Sign up for a free account on Alltius. After logging in, click on “Coach Assistants” in the left menu and then select “+Create New.” Give your assistant a name, and you’ll be ready to move on to the next step.
Step 2: Train Your AI Assistant
Now, it’s time to add sources to train your assistant. Click “+Add New Source” and choose from a list of available options. Select the data sources you want and upload the necessary files.
If you need to pull data from your software, head to the “Integrations” section in the left menu and install the required integrations.
Step 3: Test Your AI Assistant
Once your data is all set up, head to the “Playground” to test how your AI assistant works before you deploy it. If you run into any issues, our team is just a call away.
Step 4: Deploy It
The last step is deployment. Go to the “Channels” section and select “Add a New Widget.” Follow the instructions to add your brand’s touch to your AI assistant and deploy it on your website or app.
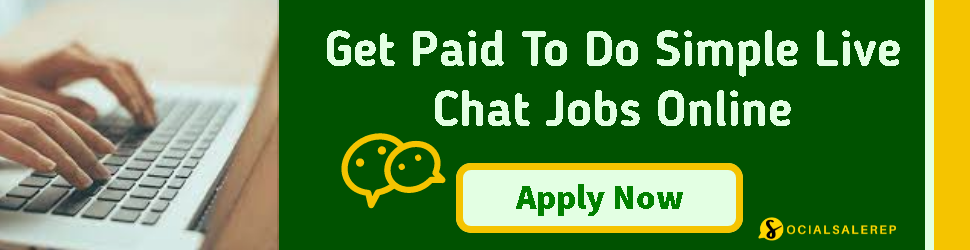
Also Read: How to Use AI for Testing Software Code?
Using Python to Build an AI Chatbot
Let’s get started by building our own Python AI chatbot! We’ve outlined the key steps for you. While this will show you how to create a basic AI chatbot, you can easily add more features to fit your needs.
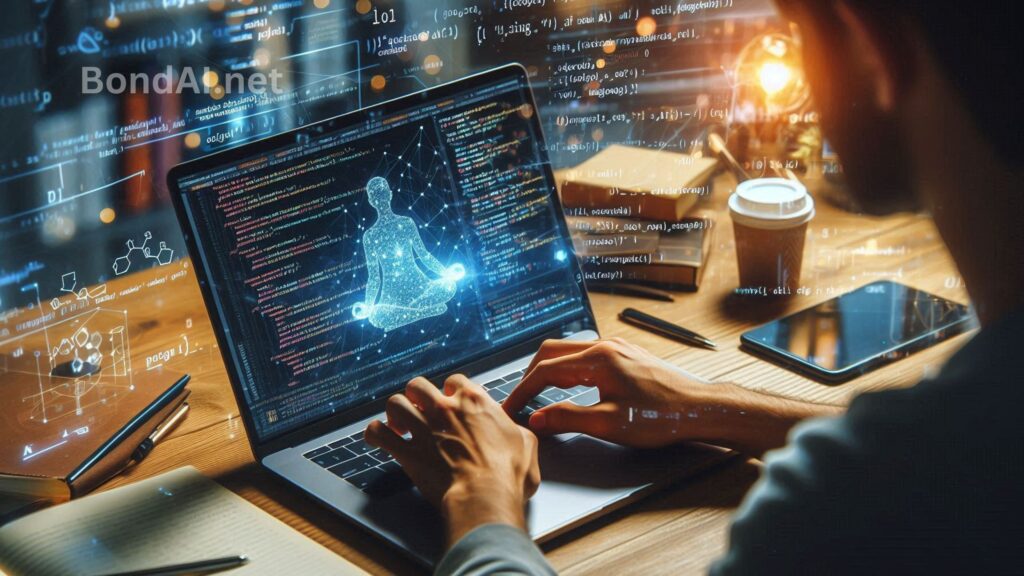
Step 1: Install Libraries
For our AI chatbot, we’ll be using the ChatterBot library. ChatterBot is a great tool that lets the chatbot learn from past conversations and inputs. To install it, just use the following pip command:
pip install chatterbot
Step 2: Import Other Libraries
Next, let’s import a couple more libraries: ChatBot and ChatterBotCorpusTrainer.
- ChatBot is used to create the chatbot instance, which allows you to interact with it.
- ChatterBotCorpusTrainer lets you train the chatbot using pre-built conversation datasets. These datasets cover different languages and topics, so you don’t have to create your own.
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
Step 3: Create Your AI Chatbot
Now, let’s create the chatbot itself. We’ll call our chatbot “Alltius,” but feel free to name yours whatever you like.
chatbot = ChatBot('Alltius')
Step 4: Train Your Chatbot with a Corpus
Next, we’ll train the chatbot using the ChatterBotCorpusTrainer. In this example, we’ll use an English language dataset. You can find many other datasets as well, depending on the language or topic you want to focus on.
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train("chatterbot.corpus.english")
Step 5: Test Your AI Chatbot
It’s time to see how our chatbot responds! Let’s test it with a simple greeting.
response = chatbot.get_response("Hello, how are you doing today?")
print(response)
Step 6: Train Your Chatbot with Custom Data
What if you want to train your chatbot with your own data? ChatterBot makes it easy using ListTrainer. With ListTrainer, you can pass a list of statements and responses, and the chatbot will learn them.
from chatterbot.trainers import ListTrainer
trainer = ListTrainer(chatbot)
trainer.train([
'How are you?',
'I am good.',
'That is good to hear.',
'Thank you.',
'You’re welcome.'
])
Step 7: Integrate Your Chatbot into a Website
Finally, if you want to integrate your chatbot into a website, you can use Flask to create a front-end for your chatbot. This way, users can interact with it through a webpage.
Here’s a simple example using Flask:
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/")
def home():
return render_template("index.html")
@app.route("/get")
def get_bot_response():
user_text = request.args.get('msg')
return str(chatbot.get_response(user_text))
if __name__ == "__main__":
app.run()
With these steps, you’ve created your own basic AI chatbot in Python! You can customize it further to suit your needs and integrate it wherever you want.
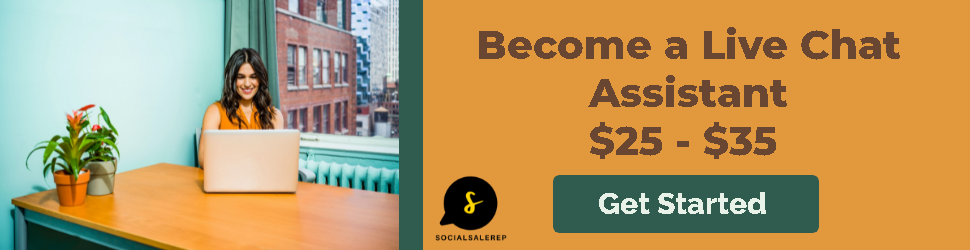
Also Read: What is the Best AI Code Editor for Developers?
Using Rasa & Rag models to build an AI chatbot

Step 1: Prepare Your Help Docs for the RAG Project
First things first: gather all the documents you’ll need to train your AI chatbot. You’ll need to clean up these docs a bit—basically, turn them into a format that’s easy for the AI to process. For this, we’ll use spaCy, a powerful library for processing natural language.
With spaCy, we can break down the text, remove unnecessary words (called “stop words”), and simplify words into their basic forms. This helps the AI focus on the most important parts of the text. Once we’ve prepped the docs, they’ll be ready for training the chatbot.
Here’s a basic example of how to use spaCy to process the docs:
import spacy
# Load spaCy's English model
nlp = spacy.load("en_core_web_sm")
# Your help docs
help_docs = """
Your help docs here.
"""
# Preprocess the text: tokenizing, removing stop words, and lemmatizing
def preprocess(text):
doc = nlp(text)
tokens = [token.lemma_ for token in doc if not token.is_stop]
return " ".join(tokens)
preprocessed_help_docs = preprocess(help_docs)
Step 2: Train the RAG Model
Now that the help docs are ready, it’s time to train the RAG (Retrieval-Augmented Generation) model. This model helps the chatbot understand the content of the documents and generate relevant responses.
We’ll use the facebook/bart-base model from the Transformers library to train the chatbot. Here’s how to do it:
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Tokenize the preprocessed help docs
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
tokenized_help_docs = tokenizer(preprocessed_help_docs, return_tensors="pt")
# Train the RAG model
model = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-base")
model.train()
loss = model(**tokenized_help_docs)
loss.backward()
optimizer.step()
This step adjusts the model’s settings so it can better understand the documents and respond correctly to user queries.
Step 3: Create a Chatbot Interface Using Rasa
Now, let’s create an interface for the chatbot. We’ll use Rasa, an open-source platform for building AI chatbots. First, set up your environment by installing Python, pip, and Rasa, then initialize your Rasa project with this simple command:
pip install rasa
rasa init
Understanding Rasa’s Key Concepts
Rasa works based on a few key concepts:
- Intents: These represent what the user wants (e.g., asking for help, making a reservation).
- Entities: These help extract important information from user queries (e.g., dates, names).
- Actions: These are what the bot does in response to a user’s query (e.g., send a message).
- Stories: These are conversations the bot can have, showing how different intents and actions connect.
For example, in your domain.yml file, you might define intents and actions like this:
intents:
- greet
- goodbye
actions:
- utter_greet
- utter_goodbye
Step 4: Create Your Chatbot
Creating the chatbot involves defining its intents, actions, and stories, and then training it. You can train the chatbot with these commands:
rasa train nlu
rasa shell
Handling more complex conversations is also possible, like asking users for multiple pieces of information or integrating external services.
Step 5: Test and Evaluate
Testing is a vital part of the process. You’ll want to use tools like rasa test for checking how well the chatbot understands user queries and how it responds. You can also use interactive learning to improve the model’s performance.
rasa interactive
rasa test core --stories data/stories.md --config config.yml
Once the chatbot is tested and ready, the next step is to deploy it, which brings it to life for real-world users.
Step 6: Deploy and Customize
Now that the chatbot is trained and tested, it’s time to deploy it. You can use Rasa’s Framework Server or deploy on a cloud platform like Heroku. Once deployed, the chatbot will be accessible to users through a simple API.
After deployment, you’ll need to do some customization, such as improving its understanding of your specific business language and adjusting its responses to suit your needs.
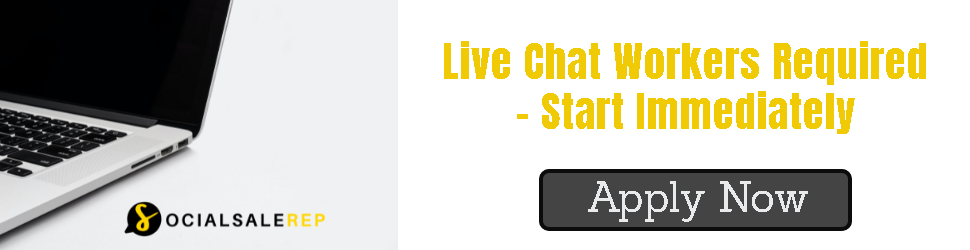
Also Read: How to Use GitHub Copilot for Coding Projects?
Challenges while building your AI Chatbot
Now that you’ve got a good idea of how to build an AI chatbot, let’s go over some of the challenges you might run into during the process:
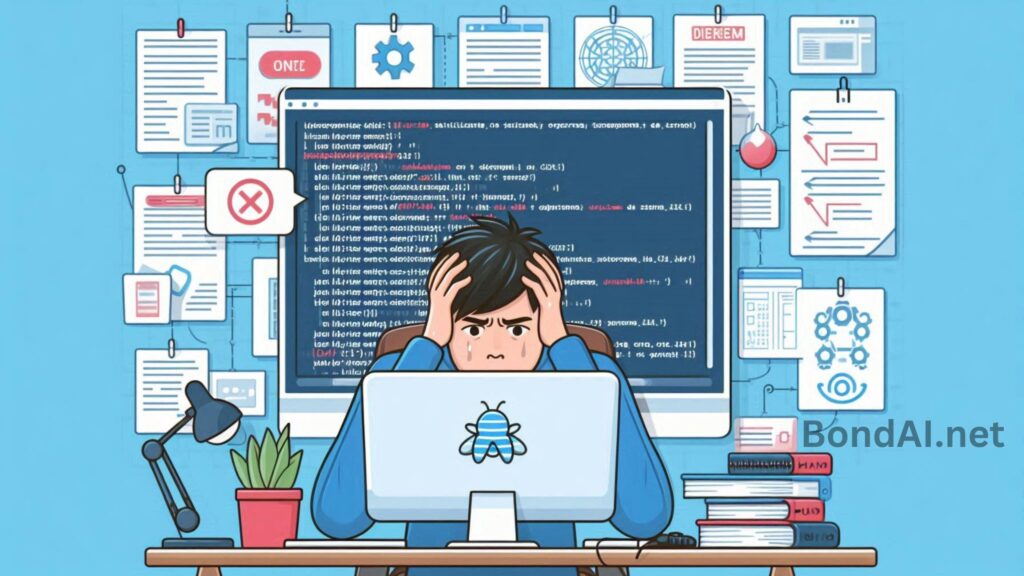
- Understanding Natural Language: One of the toughest parts is making sure the chatbot truly understands human language. This includes things like slang, idioms, and different synonyms. It takes constant tweaking to help the chatbot handle the complexities of how we communicate. Luckily, Alltius’ AI assistants are smart enough to understand the subtleties of human language, including emotions.
- Context Handling: Keeping track of a conversation over time is tricky. A chatbot needs to remember previous interactions to make current conversations feel more natural and relevant. Alltius’ AI assistants can remember past chats and use that knowledge to create a better experience for each user.
- User Intent Recognition: Figuring out what the user actually wants from their message can be tough, especially if the input is unclear. The AI chatbot must be trained on tons of different examples to accurately figure out what the user means. Alltius’ AI assistants can identify user intent with almost 99% accuracy.
- Personalization: Customizing the conversation based on a user’s preferences, past interactions, and behavior can really improve the user experience. But, it’s tricky to get it right.
- Handling Unexpected Queries: Users might ask questions or use language that the chatbot isn’t prepared for. It’s a challenge to design a chatbot that can handle unexpected inputs without disrupting the conversation. Alltius’ AI chatbots are trained to say “I don’t know” instead of offering random answers, making sure users aren’t frustrated.
- Scalability and Performance: As more users interact with the chatbot, it should still perform well without slowing down or giving inaccurate responses. Alltius’ AI chatbots are built to handle over 10,000 queries a day without breaking a sweat.
- Integration with Multiple Platforms: The chatbot needs to work seamlessly across different platforms like websites, social media, and messaging apps, each with its own set of APIs. Alltius integrates smoothly with all the major platforms.
- Data Privacy and Security: Protecting user data is essential, especially in industries like healthcare or finance. It’s important to follow strict privacy laws and standards. Alltius takes security seriously and holds certifications like SOC2, VAPT, GDPR, and ISO to make sure user data is safe.