Since OpenAI launched its GPT models, AI chatbots have been taking on all sorts of tasks, from suggesting weekly meal plans to managing customer complaints for big companies. These chatbots are proving to be a game-changer, helping automate repetitive tasks in both personal and work settings. But how do you actually build an AI chatbot using Python and NLP?
In this blog, we’ll walk you through the step-by-step process of creating a simple, conversational AI chatbot using Python and Natural Language Processing (NLP).
Understanding AI Chatbot
An AI chatbot is a smart software that mimics human conversation, either through text or voice.
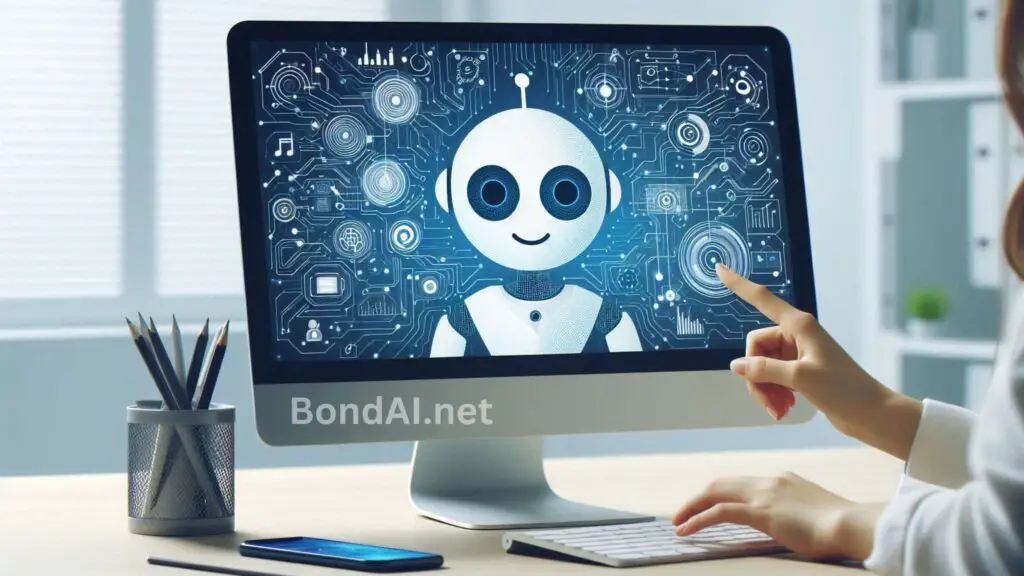
Powered by artificial intelligence, especially natural language processing (NLP), these chatbots can understand and respond to questions in a way that feels natural and human-like. They’re becoming more and more popular in areas like customer service, e-commerce, and many other industries. AI chatbots can provide 24/7 support, handle customer inquiries, and even help with sales and marketing.
What makes them even more impressive is their ability to learn from conversations. This means they get better at answering questions over time and can offer more personalized experiences. By integrating AI chatbots into business operations, companies can boost customer engagement, cut costs, and make their processes more efficient.
Different types of AI chatbots
Before you dive into building your own AI chatbot, let’s take a moment to understand the different types of chatbots.
Rule-based Chatbots
Rule-based chatbots follow a set of predefined rules, and everything they say is scripted. These chatbots are great for handling simple tasks, sticking to instructions, and providing fixed answers. However, they can’t think outside the box and struggle with complex or nuanced conversations.
AI-powered Conversational Chatbots
On the other hand, AI-powered chatbots use advanced technology to have more natural, human-like conversations. They learn from past interactions, can pull information from documents, handle conversations in multiple languages, and engage with users in a more personal way.
These chatbots are great for everything from answering basic questions to tackling more complicated product queries.
Each type of chatbot has its own strengths, so the right one for your business will depend on what you need and what you’re trying to achieve.
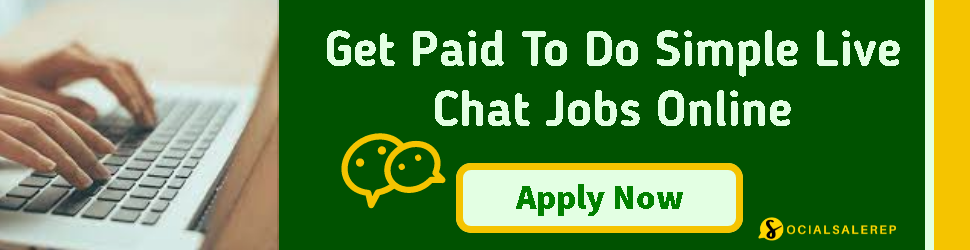
Also Read: What are the Top Trends in Machine Learning for 2025?
The smarter way to build AI chatbot : Using Alltius
Alltius is a GenAI platform that lets you create smart, secure, and accurate AI assistants without needing to code. With Alltius, you can build your own AI assistant in minutes using your own documents.
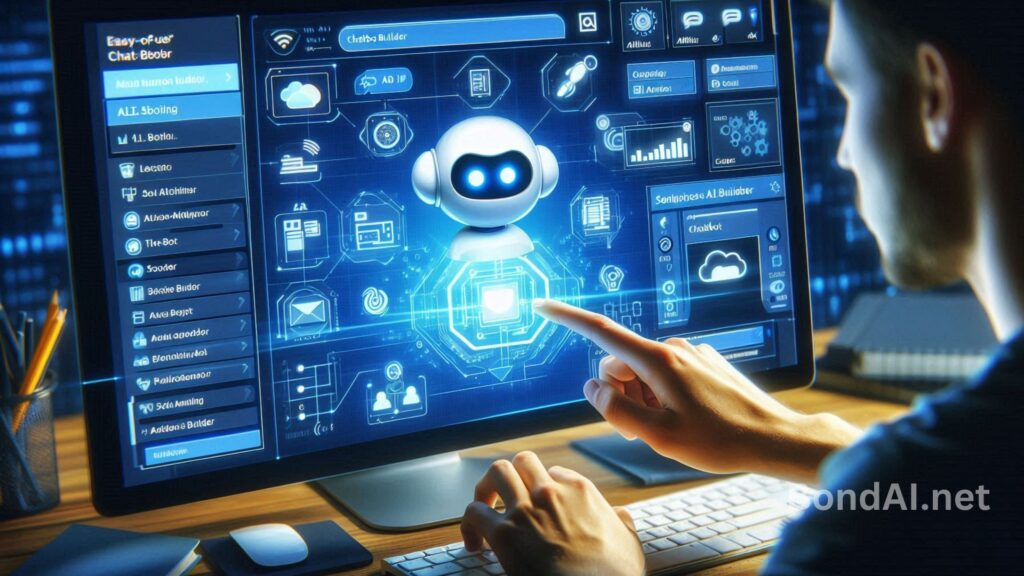
The platform is powerful, offering the widest variety of data sources to train your AI assistant. You can use PDFs, videos, emails, images, Excel files, APIs, web pages, FAQs, and more.
These assistants can do all sorts of tasks, like greeting users, answering questions, extracting information, creating pitches, drafting emails, pulling insights, and so much more. You can deploy your AI assistants on websites, Slack, Zendesk, Intercom, your product, and beyond.
Let’s walk through how easy it is to create conversational AI assistants with Alltius:
Step 1: Create Your AI Assistant
First, sign up for a free Alltius account. After logging in, click on “Coach Assistants” in the left menu and select “+Create New.” Name your assistant, and you’ll be ready to move on to the next step.
Step 2: Train Your AI Assistant
Now it’s time to add data to train your AI assistant. Click “+Add New Source” and choose from the available options like PDFs, emails, or images. Add your data, and you’re good to go! If you need to pull data from your software, simply go to the “Integrations” menu and install the required integration.
Step 3: Test the AI Assistant
Once you’ve added your data, it’s time to test your AI assistant. Head over to the Playground to chat with your assistant before you launch it. If you run into any problems, our team is just a call away.
Step 4: Deploy Your Assistant
Finally, it’s time to deploy your assistant. Go to “Channels” and click “Add a New Widget.” Follow the steps to add your brand elements and deploy the AI assistant on your website or app.
And that’s it! You’re ready to go.
Using Python to Build an AI chatbot
Let’s start by building our own Python AI chatbot! We’ve broken down the key steps for you. This guide covers a basic chatbot, but you can easily add more features to fit your needs.
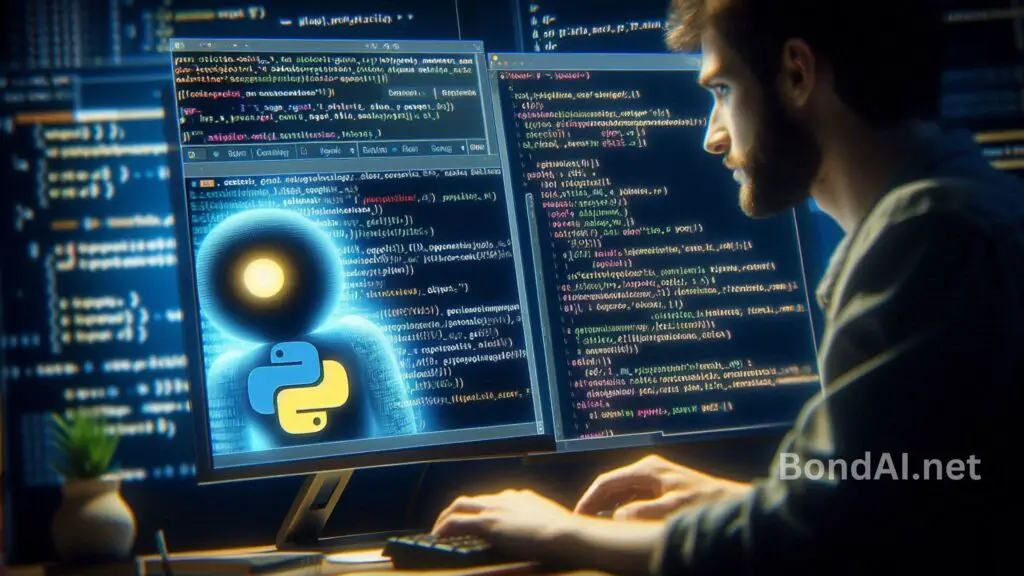
Step 1: Install Libraries
For this chatbot, we’ll use the ChatterBot library. ChatterBot is a great AI tool that helps create conversational agents that can learn from past conversations and inputs.
To get started, just run this command to install the library:
pip install chatterbot
Step 2: Import Other Libraries
Next, we’ll import some extra libraries: ChatBot
and ChatterBotCorpusTrainer
.
ChatBot
lets us create and interact with the chatbot. The ChatterBotCorpusTrainer
is used to train the chatbot using different conversational datasets.
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
Step 3: Create Your AI Chatbot
Now, let’s create the chatbot instance. You can name your chatbot whatever you like. We’ll name ours “Alltius.”
chatbot = ChatBot('Alltius')
Step 4: Train Your Python Chatbot
Now we’ll use the ChatterBotCorpusTrainer
to train our chatbot. We’ll use the English language corpus, but you can choose other languages and topics as well.
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train("chatterbot.corpus.english")
Step 5: Test Your AI Chatbot
Let’s test it! We’ll ask our chatbot a simple greeting and see how it responds.
response = chatbot.get_response("Hello, how are you doing today?")
print(response)
Step 6: Train Your Python Chatbot with Custom Data
If you want to train the chatbot with your own data, you can do that using ListTrainer
. This allows you to pass a list of questions and answers, and the chatbot will learn from them.
from chatterbot.trainers import ListTrainer
trainer = ListTrainer(chatbot)
trainer.train([
'How are you?',
'I am good.',
'That is good to hear.',
'Thank you.',
'You are welcome.'
])
Step 7: Integrate Your Python Chatbot into a Website
Now, let’s make your chatbot available on a webpage. We can use Flask to create a simple front-end for users to interact with the chatbot.
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/")
def home():
return render_template("index.html")
@app.route("/get")
def get_bot_response():
userText = request.args.get('msg')
return str(chatbot.get_response(userText))
if __name__ == "__main__":
app.run()
With this setup, your chatbot is ready to chat with users via a webpage! You can customize it even more, but this is a simple start to building your own Python AI chatbot.
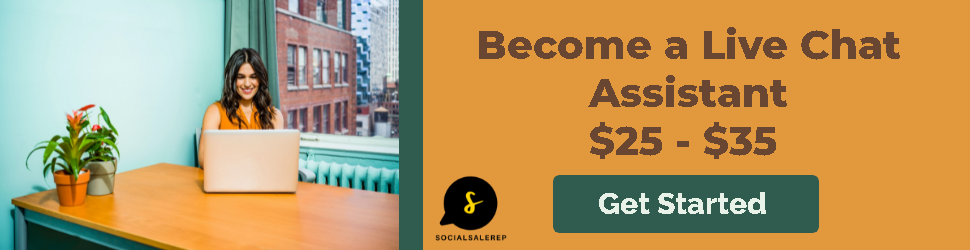
Also Read: How to Integrate AI into Mobile App Development?
Using Rasa & Rag models to build an AI chatbot
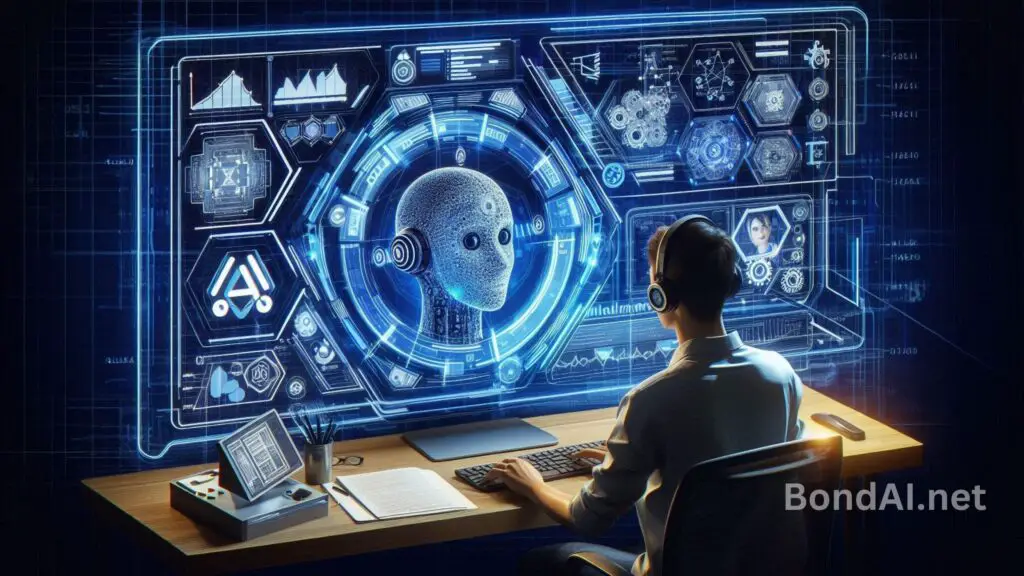
Step 1: Prepare Your Help Docs for the RAG Project
First, gather all the documents you’ll need to train your AI chatbot. These docs will need to be processed so the AI can understand them better.
Essentially, you’ll be converting raw text into a format that’s easier for the AI to work with. To do this, we’ll use spaCy, a powerful library for natural language processing (NLP).
With spaCy, we can break down the text (tokenize it), remove unnecessary words (stop words), and convert words into their basic forms (lemmatizing). This reduces the data size and helps the AI focus on important information.
Using spaCy’s features, we can clean up the help docs, making them ready for the chatbot to learn from. This preparation is key to ensuring the chatbot can understand and respond accurately to user questions.
import spacy
# Load spaCy English model
nlp = spacy.load("en_core_web_sm")
# Example help docs
help_docs = """
Your help docs here.
"""
# Tokenize, remove stop words, and lemmatize
def preprocess(text):
doc = nlp(text)
tokens = [token.lemma_ for token in doc if not token.is_stop]
return " ".join(tokens)
preprocessed_help_docs = preprocess(help_docs)
Step 2: Train the RAG Model
Next, we train the RAG (Retrieval-Augmented Generation) model, which helps the AI understand and generate responses based on the help docs.
We use the preprocessed docs to train the model. In this case, we’re using the facebook/bart-base model from the Transformers library, which is great for tasks like this.
from transformers import RagTokenizer, RagRetriever, RagSequenceForGeneration
# Tokenize the preprocessed help docs
tokenizer = RagTokenizer.from_pretrained("facebook/rag-token-base")
tokenized_help_docs = tokenizer(preprocessed_help_docs, return_tensors="pt")
# Train the RAG model
model = RagSequenceForGeneration.from_pretrained("facebook/rag-sequence-base")
model.train()
loss = model(**tokenized_help_docs)
loss.backward()
optimizer.step()
This training process fine-tunes the model so it can generate relevant responses based on the info in your docs. This is crucial for making sure the chatbot provides accurate answers to user queries.
Step 3: Create a Chatbot Interface with Rasa
Now, it’s time to set up Rasa, an open-source platform for building conversational AI applications. Rasa allows us to create a chatbot and deploy it to handle real-world conversations.
Setting up your Rasa environment:
To start, you’ll need to install Python, pip, and Rasa. Once that’s done, use the following commands to create your Rasa project:
pip install rasa
rasa init
Understand key Rasa concepts:
Rasa uses a few key concepts:
- Intents represent what the user wants.
- Entities extract important information from the user’s input.
- Actions define what the chatbot will do (like responding).
- Stories are the flow of conversation.
Here’s a basic example of defining intents and actions:
# domain.yml
intents:
- greet
- goodbye
actions:
- utter_greet
- utter_goodbye
Step 4: Build Your Chatbot
To create your chatbot, you’ll define intents, create responses, set up actions, and train the chatbot. The following commands will help you get started:
rasa train nlu
rasa shell
Handling Complex Conversations:
In real conversations, users might need to share multiple pieces of information. Rasa can handle these kinds of multi-turn conversations and integrate with external services if needed. Here’s an example of using forms for structured data:
# forms.yml
forms:
booking_form:
required_slots:
- origin
- destination
- date
Step 5: Test and Evaluate
Testing is super important to make sure your chatbot works correctly. Rasa has tools to test your NLU models and dialogue management. Here’s how you can run tests:
rasa interactive
rasa test core --stories data/stories.md --config config.yml
Deploying the Chatbot:
Once the chatbot is ready, it’s time to deploy it so users can interact with it. You can use Docker to containerize your chatbot and make it easy to deploy. Here’s how:
docker-compose up -d
rasa run --endpoints endpoints.yml
If you prefer a cloud solution, you can deploy on platforms like Heroku, which is simple to set up.
Step 6: Testing and Customizing for Production
Once your chatbot is deployed, you’ll need to do some final testing and customization. This involves refining the AI’s understanding of user queries and improving its responses.
Testing will help you find issues and improve the chatbot’s accuracy, while customization ensures it meets your specific needs.
By now, your chatbot will be ready to engage with real users and provide accurate, helpful responses based on the information it learned from the help docs.
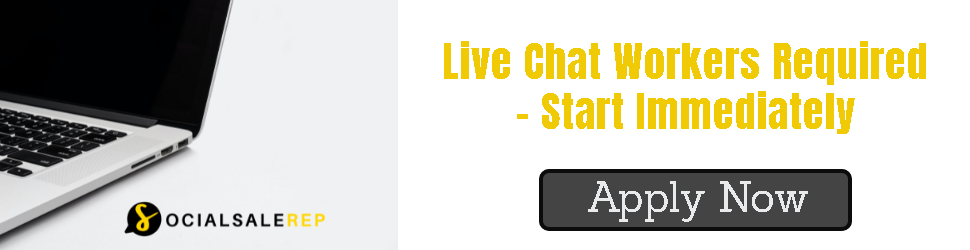
Also Read: Can AI help Debug Python Scripts?
Challenges while building your AI chatbot
Now that you have an idea of how to build an AI chatbot, let’s dive into some challenges you might face along the way:
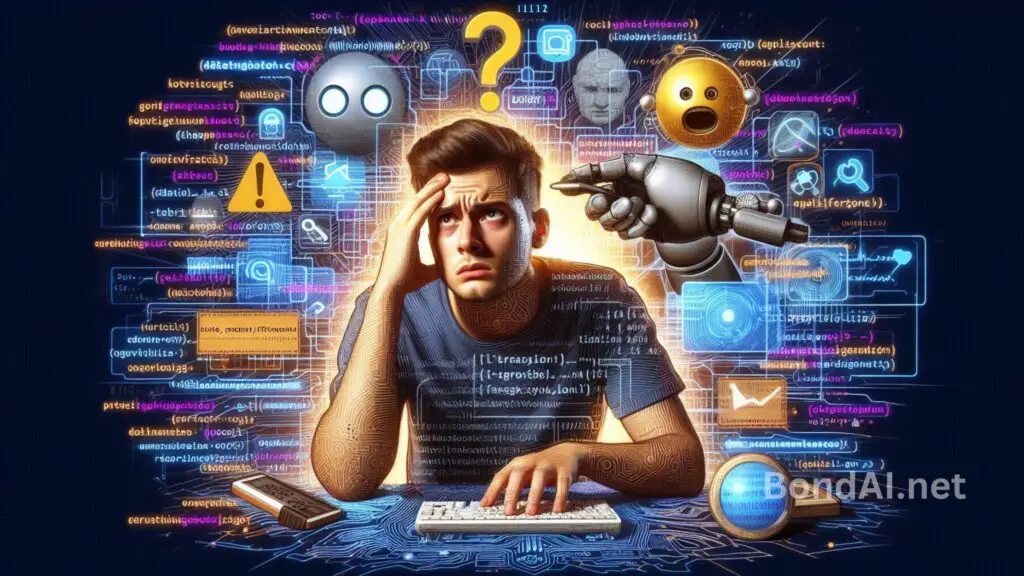
- Understanding Natural Language: One of the biggest hurdles is making sure the chatbot truly understands human language. This includes slang, idioms, and various synonyms. It takes ongoing adjustments to handle the complexity of communication. But with Alltius’ AI assistants, they’re smart enough to get the nuances of human language, including emotions.
- Context Handling: Another challenge is keeping track of the conversation context over multiple interactions. The chatbot needs to remember what was said earlier to make the current chat flow better and make sense. Alltius’ AI assistants can do this, remembering past conversations to deliver a more personalized customer experience.
- User Intent Recognition: Figuring out what the user really wants from their input can be tricky, especially if it’s unclear. The chatbot needs to be trained to handle a wide range of inputs to correctly identify what the user is asking. With Alltius, their AI assistants can recognize user intent with nearly 99% accuracy.
- Personalization: It’s important to tailor conversations to each individual, based on their preferences, history, and behavior. But it’s a tough task to do right.
- Handling Unexpected Queries: Users might ask questions or use phrases the chatbot isn’t trained for. It’s challenging to build a chatbot that handles these unexpected queries smoothly. Alltius’ AI chatbots are designed to answer with “I don’t know” instead of giving a random response that might confuse or frustrate the user.
- Scalability and Performance: As the number of users grows, the chatbot needs to scale up without slowing down or losing accuracy. Alltius’ AI chatbots are built to handle over 10,000 queries every day without a hitch.
- Integration with Multiple Platforms: The chatbot needs to work smoothly across various platforms—websites, social media, messaging apps—and deal with different APIs. Alltius can integrate with all major platforms seamlessly.
- Data Privacy and Security: Protecting user data is critical, especially in industries like healthcare and finance, where there are strict privacy laws. Alltius ensures top-level security, with certifications like SOC2, VAPT, GDPR, and ISO, making sure everything is up to standard.